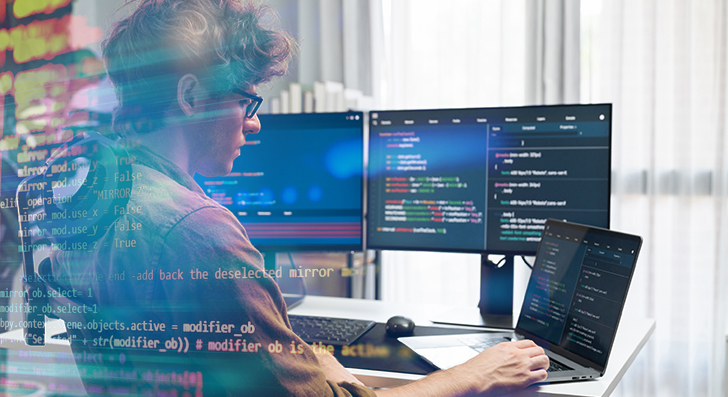
Scalability implies your software can cope with progress—much more users, additional knowledge, and even more visitors—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your prepare from the beginning. A lot of applications fall short when they increase fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As an alternative, use modular style and design or microservices. These patterns break your app into scaled-down, independent elements. Just about every module or service can scale on its own with no influencing the whole program.
Also, contemplate your databases from day a single. Will it need to have to take care of one million users or perhaps 100? Pick the ideal kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath latest ailments. Give thought to what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like concept queues or occasion-driven methods. These assist your app handle more requests with out obtaining overloaded.
Whenever you Develop with scalability in your mind, you are not just planning for achievement—you are decreasing future problems. A very well-prepared method is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases is often a crucial A part of building scalable apps. Not all databases are developed exactly the same, and utilizing the Erroneous one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your details. Could it be extremely structured, like rows inside of a table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally strong with interactions, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to take care of far more traffic and knowledge.
In case your facts is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at dealing with large volumes of unstructured or semi-structured info and will scale horizontally much more simply.
Also, consider your read through and generate patterns. Do you think you're accomplishing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which will tackle higher publish throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You might not have to have advanced scaling functions now, but selecting a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your accessibility designs. And constantly keep an eye on databases functionality while you increase.
Briefly, the appropriate databases is dependent upon your application’s construction, speed requirements, and how you anticipate it to grow. Take time to select sensibly—it’ll help save many issues later on.
Optimize Code and Queries
Quick code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complicated Alternative if an easy a single works. Keep the functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too extended to operate or employs an excessive amount of memory.
Future, examine your databases queries. These usually gradual items down more than the code by itself. Make sure each question only asks for the info you really have to have. Keep away from Find *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across substantial tables.
In the event you observe a similar info staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are fast apps. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus more traffic. If everything goes through 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes users to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts temporarily so it might be reused promptly. When consumers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it in the cache.
There's two frequent types of caching:
one. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable programs, you require applications that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and solutions as you may need them. You don’t should invest in click here hardware or guess long term capability. When targeted traffic boosts, you could increase extra resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also supply companies like managed databases, storage, load balancing, and protection instruments. You may center on setting up your application in place of taking care of infrastructure.
Containers are A different essential Device. A container packages your app and every thing it must run—code, libraries, configurations—into one particular unit. This makes it easy to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to expand without the need of limitations, start out using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your app is executing, place difficulties early, and make better choices as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just keep an eye on your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or even a support goes down, you ought to get notified right away. This aids you repair problems fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about genuine damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you keep in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your process and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge companies. Even modest applications want a solid foundation. By planning cautiously, optimizing correctly, and utilizing the proper applications, you are able to Make apps that expand effortlessly with out breaking under pressure. Get started little, Assume big, and Construct clever.